Setting up a Next.js project with Tailwind CSS
A guide to configuring your Next.js project with Tailwind CSS. We will discuss briefly the tools & frameworks that we chose, Local Project setup, Configurations, and some other nuggets.
Sat Dec 17 2022
11 min read
Mon Dec 19 2022
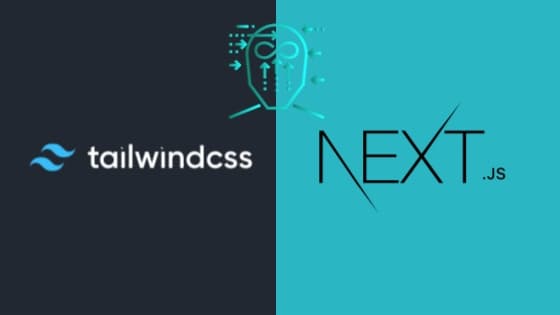
info
The below-mentioned details are mostly an extract from what I followed and
understood from the tutorial that I was following. You can find that specific
part in the tutorial here Tutorial Link - Code
setup
Want to skip the first half of this article which is slightly different that what the tutorial follows and learn things from official docs refer to this:
info
Next.js + Tailwind CSS Example This example shows how to use Tailwind
CSS
(v3.2) with Next.js. It
follows the steps outlined in the official Tailwind
docs.
I wanted to make sure to start my project with other operational things like git and so on, hence the below article also covers those aspect which are not covered in the tutorial in the beginning.
Introduction
Next.js is a popular framework for building server-rendered React applications. It provides a set of utilities and conventions that make building scalable and performant apps easy. Tailwind CSS is a utility-first CSS framework that allows you to build custom designs without writing custom CSS. Together, Next.js and Tailwind CSS make it easy to build fast and maintainable web applications.
Prerequisites
Before you get started, you'll need to have the following tools installed on your machine:
- Node.js (version 10 or higher)
- npm (comes with Node.js)
- npx (comes with npm)
Note this is the Frontend setup for this project, Backend will be covered in the next article.
Project setup
To set up a new Next.js with Tailwind CSS project, open a terminal and navigate to the directory where you want to create your project. Then, run the following command:
consolenpx create-next-app my-project -e with-tailwindcss
This will create a new Next.js project in a directory called
my-project
.OR, if you already have the project directory then use below command
consolenpx create-next-app -e with-tailwindcss ./
Configuration
If you have installed and initiated the NextJS project without the Tailwind CSS then follow the below steps, else look at this part in the Tutorial CSS-Setup
!!! warning Mistakes
Since I messed up following a neat instruction and was over-enthusiastic about following independent framework documentation decided to set up the project in a very different way. Learning: Try things out as mentioned by the tutor, if they don't work then explore. But this was my own way of unlearning and relearning.
!!!
To configure Tailwind CSS in your Next.js project, you'll need to create a configuration file. Run the following command to generate a default configuration file:
consolenpx tailwindcss init
This will install the Tailwind CSS package and its dependencies.
Next, create a new file called
tailwind.config.js
in the root of your project and add the following code:jsmodule.exports = { content: [ "./pages/**/*.{js,ts,jsx,tsx}", "./components/**/*.{js,ts,jsx,tsx}", "./app/**/*.{js,ts,jsx,tsx}", ], theme: { extend: {}, }, variants: {}, plugins: [], };
This is the configuration file for Tailwind CSS. You can use it to customize the default styles and add new styles to your project. I did play around to customize things for my needs like background gradients color palette through out the project which was a great learning.
**Adding the CSS to your project**
If you have followed the tutorial then you will understand to add things to the
global.scss
file under the styles folder.I am not that experienced when it comes to CSS or tailwind but learn a lot while doing this project. So far added the styles by looking at the documentation and have slowly started to realize that there is a much better way to define, organize and maintain the UI design system using TailwindCSS and its said configuration.
!!! tip Learning
I just followed the tutor so that I don't make mistakes to try very different things on the CSS front.
!!!
For new developers: Key groundwork
!!! tip Learning
While reviewing this article realized that the below content can be a series of its own, but decided to keep the below content as it is, will be adding the Operation aspect of development things related articles as a backlog for now
!!!
Other helpful things when setting up a new project for an individual or also for people in organizations where you don’t follow it, this might still be very useful are:
Adding Git and GitHub templates
It's a good idea to set up your project with version control using Git. To do this, navigate to the root of your project in a terminal and run the following commands:
consolegit init git add . git commit -m "Initial commit"
These commands initialize a new Git repository, add all of the files in your project to it, and create an initial commit.
Next, create a new repository on GitHub and follow the instructions to push your local repository to the remote repository on GitHub.
To make it easier to manage your repository, you can set up templates for issues and pull requests. To do this, create a new file called
.github/ISSUE_TEMPLATE.md
in the root of your project and add the following content:md## Description [Description of the issue] ## Steps to Reproduce 1. [Step 1] 2. [Step 2] 3. [Step 3] ## Expected Behavior [What you expected to happen] ## Actual Behavior [What actually happened] ## Additional Information [Any additional information, such as screenshots or error messages]
This is a template for creating new issues in your repository. You can customize it to fit your needs.
To create a template for pull requests, create a new file called
.github/PULL_REQUEST_TEMPLATE.md
and add the following content:md## Description [Description of the changes being made] ## Related Issues [List of related issues] ## Changes Made - [Change 1] - [Change 2] - [Change 3] ## Checklist - [ ] I have tested these changes - [ ] I have updated the documentation (if necessary)
This template helps you keep track of the changes being made in a pull request and ensures that all necessary tasks have been completed before merging the pull request.
!!! note
the above functionality or setup might vary based on what type of git versioning system you might be using.
!!!
Adding a .gitignore file
To prevent unnecessary files from being added to your Git repository, you can create a
.gitignore
file. This file specifies which files and directories should be ignored by Git.To create a
.gitignore
file, create a new file in the root of your project called .gitignore
and add the following content:node_modules/ out/ .env .DS_Store # Or any other files
This will ignore the
node_modules
, out
, .env
, and .DS_Store
directories and files. You can add any other files or directories that you don't want to track with Git.This is a nice website to generate gitignore files based on your setup:
Setting up IDE:
IDE - stands for Integrated development environmentI, my choice for now would be Visual Studio Code
Visual Studio Code (VS Code) is a popular code editor that has great support for Next.js and JavaScript. To set up VS Code for your project, follow these steps:
- Install VS Code if you haven't already.
- Open your project in VS Code.
- Install the following extensions:
- ESLint
- Prettier - Code Formatter
- Open the VS Code settings (File > Preferences > Settings or by pressing
Ctrl + ,
) and add the following to your settings:
js"editor.formatOnSave": true, "eslint.autoFixOnSave": true, "eslint.validate": [ "javascript", "javascriptreact", { "language": "typescript", "autoFix": true }, { "language": "typescriptreact", "autoFix": true } ]
This will enable automatic formatting and linting when you save a file.
If you are greeted with latest VSCode UI for settings then search for above keys and set it to state mentioned above
Setting up a changelog
A changelog is a file that lists the changes made to a project over time. It's a good practice to maintain a changelog for your project because it helps keep track of the changes that have been made and makes it easier for users and contributors to understand the history of the project.
To set up a changelog for your Next.js project with Tailwind CSS, follow these steps:
- Create a new file in the root of your project called
CHANGELOG.md
. - Add the following content to the file:
md# Changelog All notable changes to this project will be documented in this file. The format is based on [Keep a Changelog](https://keepachangelog.com/en/1.0.0/), and this project adheres to [Semantic Versioning](https://semver.org/spec/v2.0.0.html). ## [Unreleased] - Initial release
This is the basic structure of a changelog file. It includes a header, the format of the changelog, and an initial entry for the initial release of the project.
- As you make changes to your project, add new entries to the changelog to document the changes. Each entry should include a description of the change, the type of change (e.g. bug fix, feature, etc.), and the issue or pull request that the change was related to.
Here's an example of a changelog entry:
md## [1.0.0] - 2021-01-01 ### Added - Added a new feature to the application - Added a new component to the application ### Fixed - Fixed a bug that caused the application to crash - Fixed a bug that caused incorrect data to be displayed ### Changed - Improved the performance of the application ### Closed - Closed issue #123: Add new feature to the application - Closed pull request #456: Fix application crash bug
- When you're ready to release a new version of your project, update the
Unreleased
section with the changes that have been made since the last release and add a new section for the current release.
Here's an example of a changelog with multiple releases:
md# Changelog All notable changes to this project will be documented in this file. The format is based on [Keep a Changelog](https://keepachangelog.com/en/1.0.0/), and this project adheres to [Semantic Versioning](https://semver.org/spec/v2.0.0.html). ## [Unreleased] - Added a new feature to the application - Added a new component to the application ## [1.0.0] - 2021-01-01 ### Added - Initial release of the application ## [1.0.1] - 2021-01-15 ### Fixed - Fixed a bug that caused the application to crash - Fixed a bug that caused incorrect data to be displayed ### Changed - Improved the performance of the application ### Closed - Closed issue #123: Add new feature to the application - Closed pull request #456: Fix application crash bug
By maintaining a changelog for your project, you can keep track of the software or a project’s growth or evolution. Also for anyone interested too this acts as a timeline for all the work that has gone in.
Best practices for folder structure for a NextJS project with Tailwind CSS
There are several best practices for organizing the folder structure of a Next.js project with Tailwind CSS. Here are a few suggestions:
- Create a
src
directory at the root of your project to store all of your source code. This directory should contain the following subdirectories:components
: This directory should contain your reusable React components.css
: This directory should contain your CSS files, including your mainindex.css
file and any other CSS files you might have.pages
: This directory should contain your Next.js pages.public
: This directory should contain any static assets that you want to be publicly accessible, such as images or fonts.
- Keep your pages and components organized by creating subdirectories for different sections or features of your application. For example, you might have a
/pages/auth
directory for your authentication pages and a/components/forms
directory for your form components. - If you have a lot of pages or components, you might want to create a
/pages/shared
or/components/shared
directory for shared elements that are used across multiple pages or components. - Keep your CSS organized by creating separate files for different sections or features of your application. For example, you might have a
/css/header.css
file for your header styles and a/css/footer.css
file for your footer styles. - Consider creating a
/out
directory to store the built CSS files. This can help keep your source code directory clean and separate from the built assets.
Overall, the key is to keep your code organized and easy to navigate. This will make it easier to maintain and update your project over time.
!!! abstract Disclaimer:
I personally didn't follow exactly what is stated above, as I was following the tutorial I decided to follow the tutor on overall concepts and decided to stick with it. The main fundamental difference is that we didn't nest things under the
src/
folder
!!!Conclusion
In this article, you learned how to set up a Next.js project with Tailwind CSS. You also learned how to set up version control with Git and GitHub, create templates for issues and pull requests, set up a changelog, and configure VS Code for your project. With these tools and practices in place, you're ready to start building your Next.js application with Tailwind CSS.